Type checking occurs in python in many ways and is useful in various contexts such as in Algorithms. For example, assume we had the following simple function:
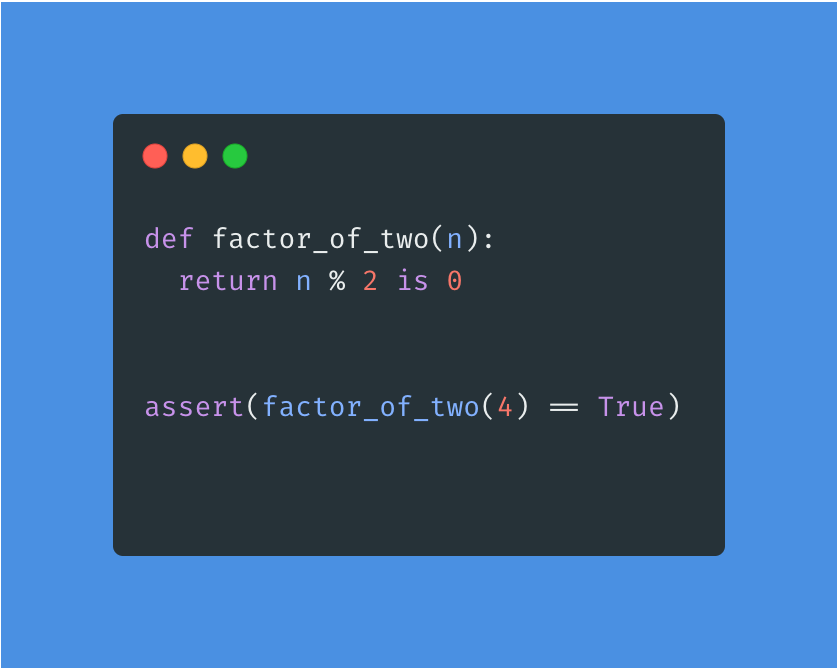
We could assume that the function will work correctly. However, we can try breaking the code like this:
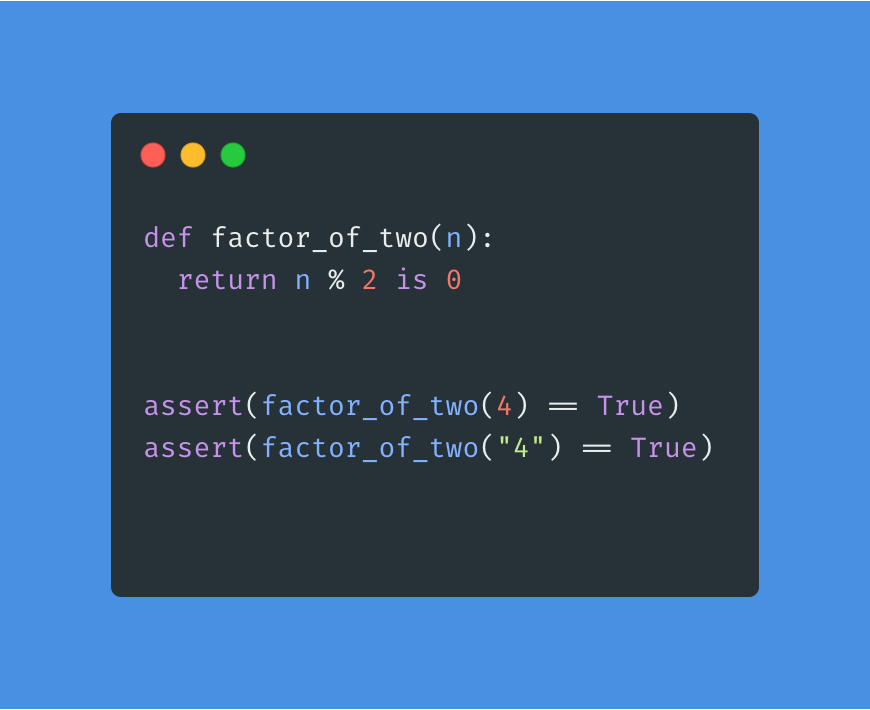
Now the code breaks. Since we are expecting a number, we instead get a string value. Although this example is stupid simple, it shows just how important type checking in python really is. We can amend the code using the isinstance() function defined with these parameters:
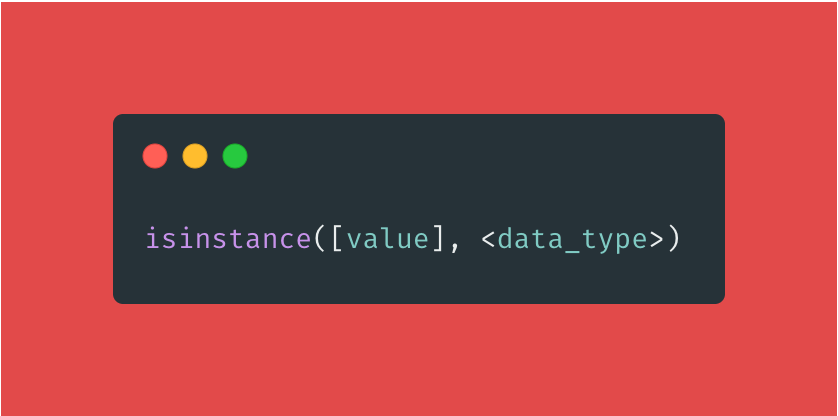
We can compare the value and data type in order to create an edge case check for our function above like below:
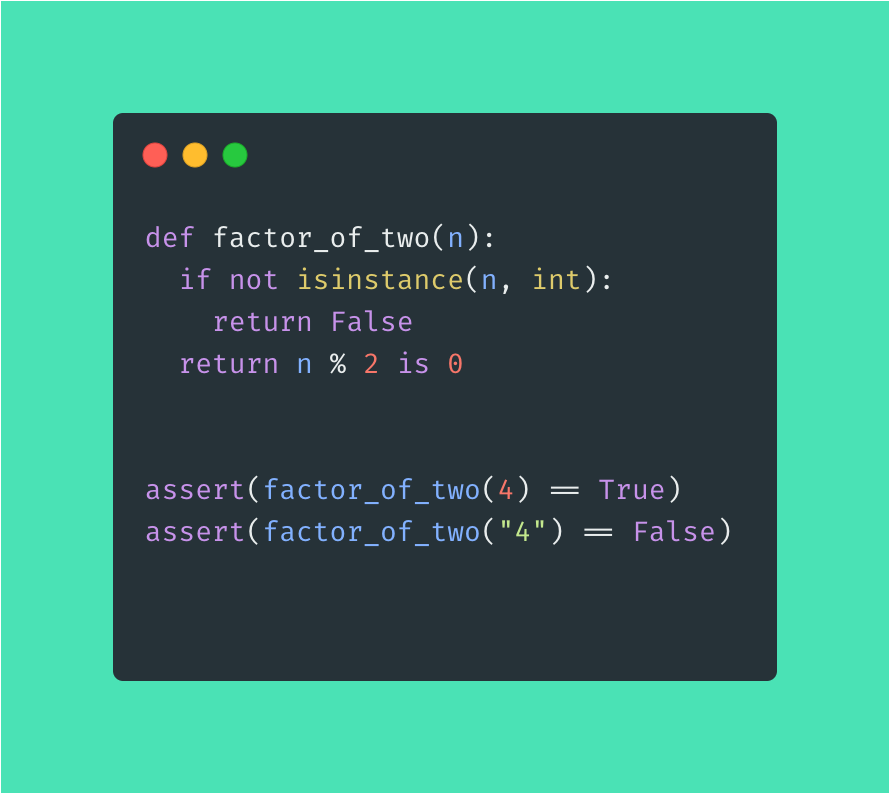
Booya! Problem solved. Question; what else could you optimize in the function in order to clear out other non-obvious edge cases?
How is it different from type()?
That’s a great question! Although they tend to both resolve a type based on the value passed, isinstance() comes out on top. Assuming we define a class and a subclass as seen below:
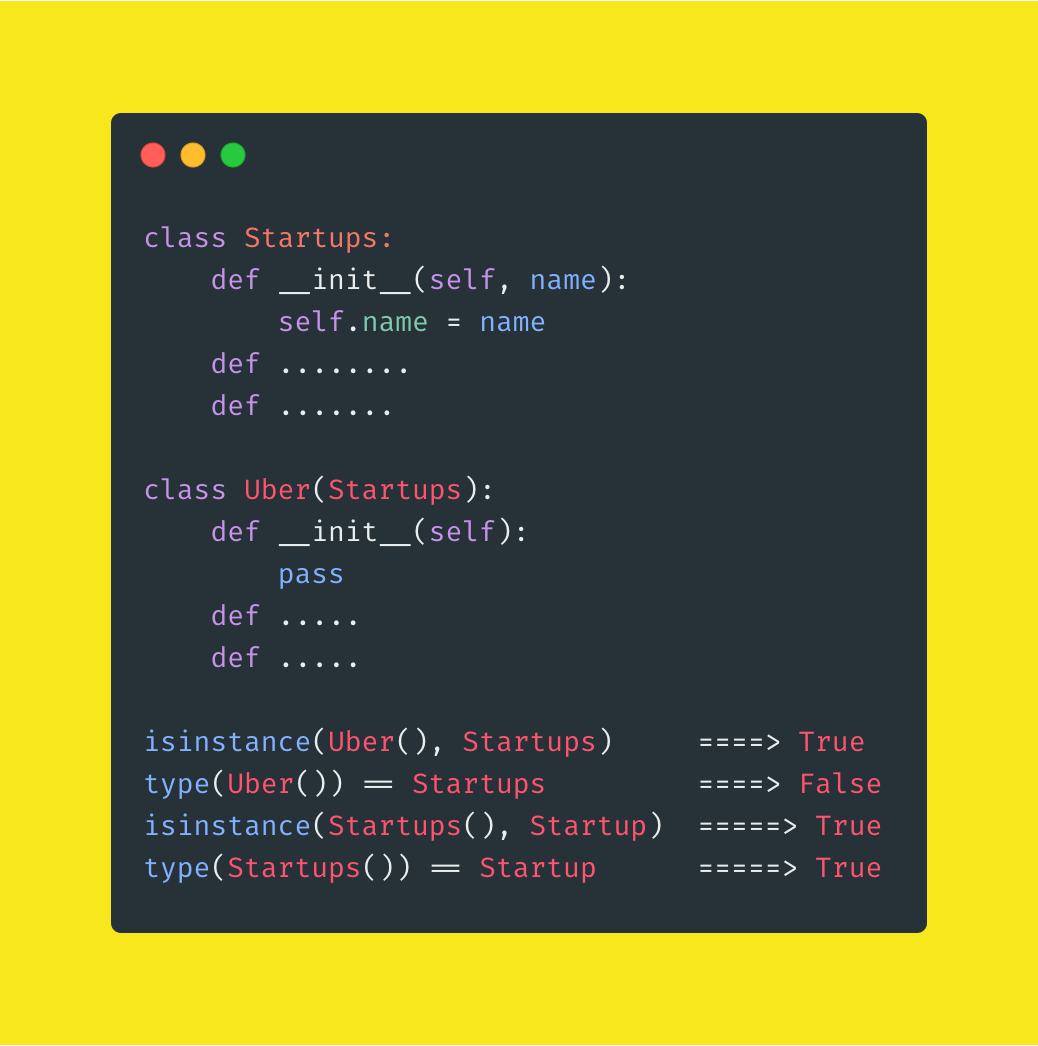
(Source: https://stackoverflow.com/questions/1549801/what-are-the-differences-between-type-and-isinstance )
isinstance() is able to detect subclasses as well. You can actually confirm this by checking out the tests for is instance on Github here:
https://github.com/python/cpython/blob/1c56f8ffad44478b4214a2bf8eb7cf51c28a347a/Lib/test/test_isinstance.py
So what should I use? isinstance() or type?
Personally, I would prefer to use isinstance() not only because it makes a deeper comparison, but also because I prefer the syntax better 😀.
What do you think?
If you have any thoughts, please feel free to let me know!